C++ IN INHERITENCE
In C++, inheritance is a process in which one object acquires all the properties and behaviors of its parent object automatically. In such way, you can reuse, extend or modify the attributes and behaviors which are defined in other class.
In C++, the class which inherits the members of another class is called derived class and the class whose members are inherited is called base class. The derived class is the specialized class for the base class.
ADVANTAGE OF C++ INHERITANCE;
TYPES OF INHERTANCE;
C++ supports five types of inheritance:
- Single inheritance
- Multiple inheritance
- Hierarchical inheritance
- Multilevel inheritance
- Hybrid inheritance
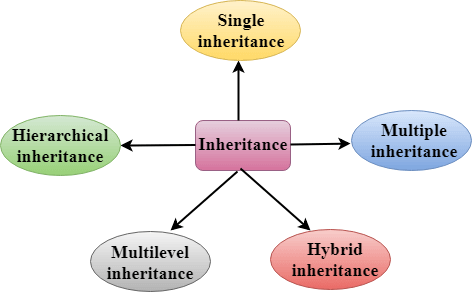
DERIVED CLASSES;
A Derived class is defined as the class derived from the base class.
The Syntax of Derived class:
Where,
derived_class_name: It is the name of the derived class.
visibility mode: The visibility mode specifies whether the features of the base class are publicly inherited or privately inherited. It can be public or private.
base_class_name: It is the name of the base class.
- When the base class is privately inherited by the derived class, public members of the base class becomes the private members of the derived class. Therefore, the public members of the base class are not accessible by the objects of the derived class only by the member functions of the derived class.
- When the base class is publicly inherited by the derived class, public members of the base class also become the public members of the derived class. Therefore, the public members of the base class are accessible by the objects of the derived class as well as by the member functions of the base class.
Note:
- In C++, the default mode of visibility is private.
- The private members of the base class are never inherited.
C++ SINGLE INHERITANCE;
Single inheritance is defined as the inheritance in which a derived class is inherited from the only one base class.
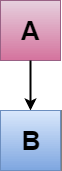
Where 'A' is the base class, and 'B' is the derived class.
C++ SINGLE LEVEL INHERITANCE EXAMPLE:
INHERITING FIELDS
When one class inherits another class, it is known as single level inheritance. Let's see the example of single level inheritance which inherits the fields only.
Output:
Salary: 60000
Bonus: 5000
In the above example, Employee is the base class and Programmer is the derived class.
HOW TO MAKE A PRIVATE MEMBER INHERITANCE;
The private member is not inheritable. If we modify the visibility mode by making it public, but this takes away the advantage of data hiding.
C++ introduces a third visibility modifier, i.e., protected. The member which is declared as protected will be accessible to all the member functions within the class as well as the class immediately derived from it.
Visibility modes can be classified into three categories:
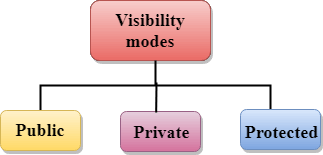
- Public: When the member is declared as public, it is accessible to all the functions of the program.
- Private: When the member is declared as private, it is accessible within the class only.
- Protected: When the member is declared as protected, it is accessible within its own class as well as the class immediately derived from it.
VISIBILITY OF INHERITANCE MAMBERS;
Base class visibility | Derived class visibility | ||
---|---|---|---|
Public | Private | Protected | |
Private | Not Inherited | Not Inherited | Not Inherited |
Protected | Protected | Private | Protected |
Public | Public | Private | Protected |
C++ MULTILEVEL INHERITANCE;
Multilevel inheritance is a process of deriving a class from another derived class.
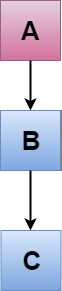
C++ MULTI LEVEL INHERITANCE EXAMPLE;
When one class inherits another class which is further inherited by another class, it is known as multi level inheritance in C++. Inheritance is transitive so the last derived class acquires all the members of all its base classes.
Let's see the example of multi level inheritance in C++.
Output:
Eating... Barking... Weeping...
C++ MULTIPLE INHERITANCE;
Multiple inheritance is the process of deriving a new class that inherits the attributes from two or more classes.
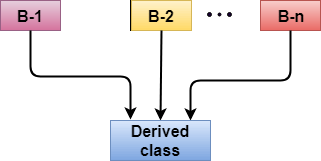
Syntax of the Derived class:
Let's see a simple example of multiple inheritance.
Output:
The value of a is : 10
The value of b is : 20
Addition of a and b is : 30
In the above example, class 'C' inherits two base classes 'A' and 'B' in a public mode.
C++ HYBIRD INHERITANCE;
Hybrid inheritance is a combination of more than one type of inheritance.
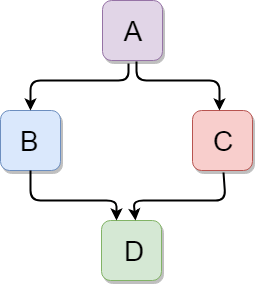
Let's see a simple example:
Output:
Enter the value of 'a' : 10 Enter the value of 'b' : 20 Enter the value of c is : 30 Multiplication of a,b,c is : 6000
C++ HIERARCHICAL INHERITANCE;
Hierarchical inheritance is defined as the process of deriving more than one class from a base class.
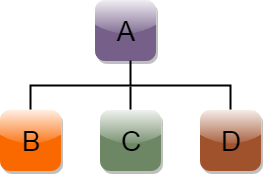
Syntax of Hierarchical inheritance:
Let's see a simple example:
Output:
Enter the length and breadth of a rectangle:
23
20
Area of the rectangle is : 460
Enter the base and height of the triangle:
2
5
Area of the triangle is : 5
Comments
Post a Comment